Shell中函数
时间:2016-06-24 00:53 来源:linux.it.net.cn 作者:IT
一 ,shell函数的注意事项
Here is a review of some of the important rules about using functions.
下面是使用函数的一些重要规则
1,The shell determines whether you are using an alias, a function, a built-in command, or an executable program (or script) found on the disk. It looks for aliases first, then functions, built-in commands, and executables last.
Shell判断你是在使用别名、函数、内建命令或者磁盘上的可执行程序时的顺序是,别名、函数、内建命令最后是可执行程序
2,A function must be defined before it is used.
函数必须定义在前,使用在后。
3,The function runs in the current environment; it shares variables with the script that invoked it, and lets you pass arguments by assigning them as positional parameters. Local variables can be created within a function by using the local function.
函数在当前环境中运行,共享当前脚本触发的环境变量,你可以传递参数,也可以在函数内部创建局部环境变量。
4,If you use the exit command in a function, you exit the entire script. If you exit the function, you return to where the script left off when the function was invoked.
假如你在函数中使用exit命令,你将退出整个脚本,加入你想退出函数,你将返回到函数被触发的地方
5,The return statement in a function returns the exit status of the last command executed within the function or the value of the argument given.
函数中的返回声明返回函数中最后一条命令的退出状态或者给定的参数值
6,Functions can be exported to subshells with the export –f built-in command.
7,To list functions and definitions, use the declare –f command. To list just function names, use declare –F .[5]
可以使用declare -f命令列出函数和定义,使用declare -F列出函数名称。
[5] Only on bash versions 2.x.只在2.x以上版本有效
8,Traps, like variables, are global within functions. They are shared by both the script and the functions invoked in the script. If a trap is defined in a function, it is also shared by the script. This could have unwanted side effects.
Traps,像变量一样,在函数内部是全局的。他们被脚本和脚本内部触发的函数共享。假如trap是在脚本你定义的,他也是被脚本共享的,这可能导致一些非预期的负面影响
9,If functions are stored in another file, they can be loaded into the current script with the source or dot command假如函数在另外一个文件中,他可以被加载到当前脚本中使用source或者dot 命令.
10,Functions can be recursive; that is, they can call themselves. There is no limit imposed for the number of recursive calls.
函数可以使递归的,即,函数可以自己调用自己。递归调用的次数没有限制
二,shell函数的定义和使用
Format
function function_name { commands ; commands; }
Example 14.55.
function dir { echo "Directories: ";ls -l|awk '/^d/ {print $NF}'; }
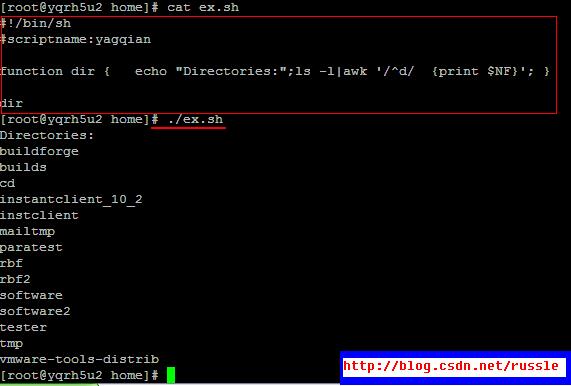
EXPLANATION
The keyword function is followed by the name of the function dir. (Sometimes empty parentheses follow the function name, but they are not necessary.) The commands within the curly braces will be executed when dir is typed. The purpose of the function is to list only the subdirectories below the present working directory. The spaces surrounding the first curly brace are required.
关键字function之后紧随的是函数名称dir(有时函数名称后是空括号)大括号内的命令在调用dir时将被执行。这个函数的作用列出当前工作目录的子目录。第一个大括号的空格是必须的
三,shell函数的参数
Function Arguments and the Return Value
函数的参数和返回值
Because the function is executed within the current shell, the variables will be known to both the function and the shell. Any changes made to your environment in the function will also be made to the shell.
函数是在当前shell中执行的,变量是函数和shell都知道的,任何对环境变量的改变shell都会知道的
Arguments 参数
Arguments can be passed to functions by using positional parameters. The positional parameters are private to the function; that is, arguments to the function will not affect any positional parameters used outside the function. See Example 14.56.
可以给函数传递参数,参数通过位置来区分。位置对函数是私有的,位置只对该函数有效,不影响该函数外的,参看例子
The Built-In local Function
To create local variables that are private to the function and will disappear after the function exits, use the built-in local function. See Example 14.57.
The Built-In return Function
The return command can be used to exit the function and return control to the program at the place where the function was invoked. (Remember, if you use exit anywhere in your script, including within a function, the script terminates.) The return value of a function is really just the value of the exit status of the last command in the script, unless you give a specific argument to the return command. If a value is assigned to the return command, that value is stored in the ? variable and can hold an integer value between 0 and 256. Because the return command is limited to returning only an integer between 0 and 256, you can use command substitution to capture the output of a function. Place the entire function in parentheses preceded by a $ (e.g., $(function_name)), or traditional backquotes to capture and assign the output to a variable just as you would if getting the output of a UNIX command.
Example 14.56.
(Passing Arguments)
(The Script)
#!/bin/bash
# Scriptname: checker
# Purpose: Demonstrate function and arguments
1 function Usage { echo "error: $*" 2>&1; exit 1; }
2 if (( $# != 2 ))
then
3 Usage "$0: requires two arguments"
fi
4 if [[ ! ( -r $1 && -w $1 ) ]]
then
5 Usage "$1: not readable and writable"
fi
6 echo The arguments are: $*
< Program continues here >
(The Command Line and Output)
$ checker
error: checker: requires two arguments
$ checker file1 file2
error: file1: not readable and writable
$ checker filex file2
The arguments are filex file2
EXPLANATION
1 The function called Usage is defined. It will be used to send an error message to standard error (the screen). Its arguments consist of any string of characters sent when the function is called. The arguments are stored in $*, the special variable that contains all positional parameters. Within a function, positional parameters are local and have no effect on those positional parameters used outside the function.
定义名称为Usage的函数,他将向标准错误(屏幕)输出错误信息。他的参数是调用时的任何字符串。参数存储在$*,这个特殊变量包含了所有的参数。在函数内部,位置参数是局部的,对函数外的没有任何影响。
2 If the number of arguments being passed into the script from the command line does not equal 2, the program branches to line 3.
假如从命令行传递给脚本的参数不等于2个,程序跳转到第三行
3 When the Usage function is called, the string $0: requires two arguments is passed to the function and stored in the $* varable. The echo statement will then send the message to standard error and the script will exit with an exit status of 1 to indicate that something went wrong.[a]
当Usage被调用时,字符串$0:要求传递两个参数,存储在$*变量中,echo命令将其发送到标准错误输出,脚本退出,退出的状态时1,暗示出错了
[a] With the old test form, the expression is written if [ ! /( -r $1 -a -w $1 /) ].
4, 5 If the first argument coming into the program from the command line is not the name of a readable and writable file, the Usage function will be called with $1: not readable and writable as its argument.
假如从命令行传递的第一个参数不是一个可读可写的文件名,Usage将被调用使用$1,不可读可写作为他的参数
6 The arguments coming into the script from the command line are stored in $*. This has no effect on the $* in the function.
四 ,shell函数的返回值
函数的返回值
#!/bin/bash
# Scriptname: do_increment
increment () {
local sum; # sum is known only in this function
let "sum=$1 + 1"
return $sum # Return the value of sum to the script
}
echo –n "The sum is "
increment 5 # Call function increment; pass 5 as a
# parameter; 5 becomes $1 for the increment function
echo $? # The return value is stored in $?
echo $sum # The variable "sum" is not known here
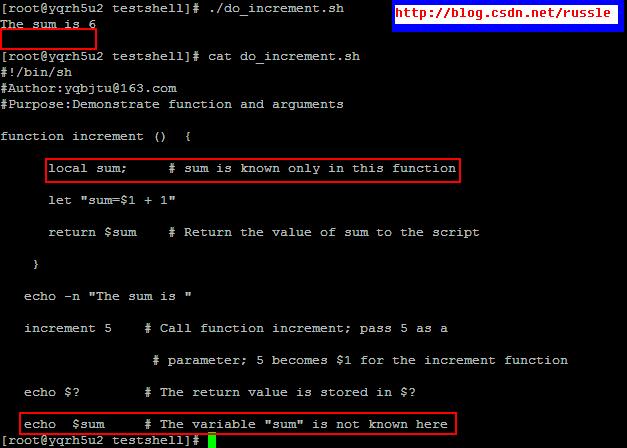
返回值例子二
#!/bin/bash
# Scriptname: do_square
function square {
local sq # sq is local to the function
let "sq=$1 * $1"
echo "Number to be squared is $1."
echo "The result is $sq "
}
echo "Give me a number to square. "
read number
value_returned=$(square $number) # Command substitution
echo "$value_returned"
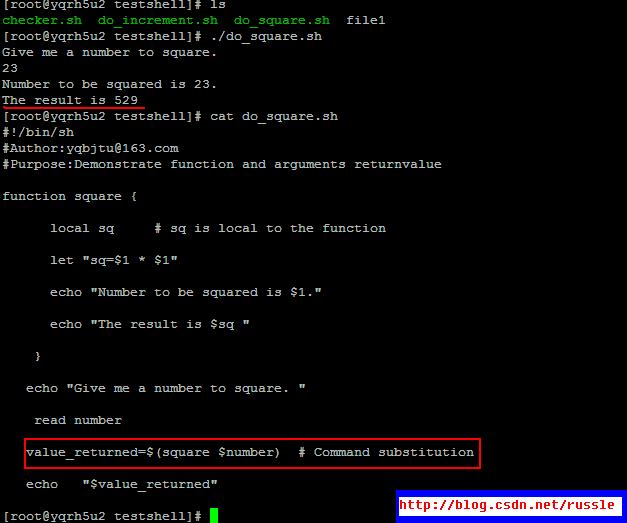
五,Shell函数和source命令
将函数定义在一个文件中,通过source命令或者。命令,部署到当前的shell中,需要时可以直接在当前shell中执行
文件myfunciton
go() { # This file contains two functions
cd $HOME/bin/prog
PS1='`pwd` > '
ls
}
greetings() { echo "Hi $1! Welcome to my world." ; }
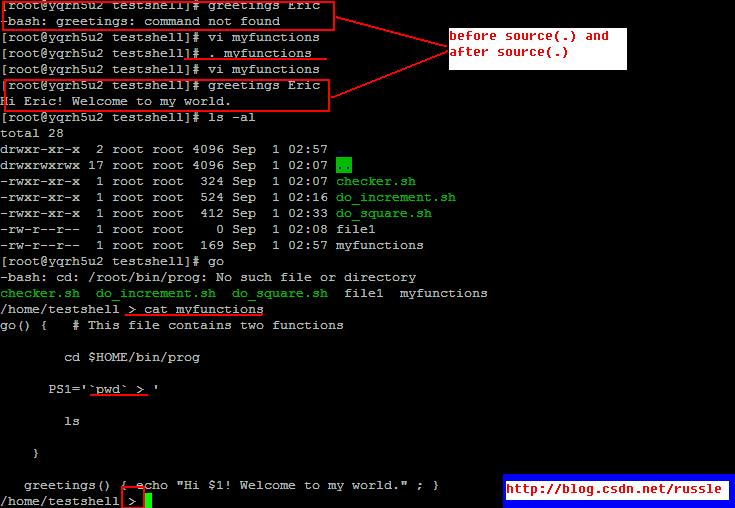
(责任编辑:IT)
一 ,shell函数的注意事项
Here is a review of some of the important rules about using functions. 二,shell函数的定义和使用
Format
四 ,shell函数的返回值 函数的返回值 #!/bin/bash # Scriptname: do_increment
increment () {
返回值例子二 #!/bin/bash # Scriptname: do_square
function square {
五,Shell函数和source命令 将函数定义在一个文件中,通过source命令或者。命令,部署到当前的shell中,需要时可以直接在当前shell中执行 文件myfunciton
go() { # This file contains two functions
(责任编辑:IT) |